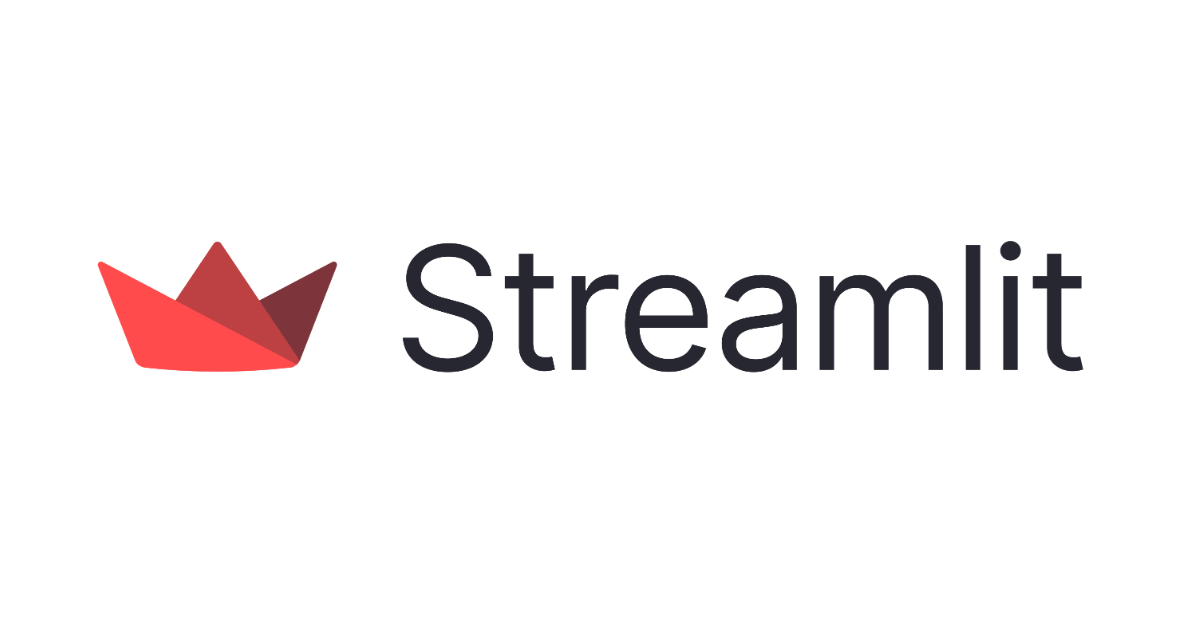
『30 Days of Streamlit』を3日でやってみた #2 (Day11〜Day20) #30DaysOfStreamlit
"『30 Days of Streamlit』を3日でやってみた"シリーズ第2弾、11日目から20日目の実践内容です。
第1弾(01日目〜10日目)の内容はこちら:
目次
実践記録(Day11〜Day20)
Day 11: st.multiselectの実装
複数選択ウィジェットを表示するst.multiselect
の実践。
実装コード:
import streamlit as st st.header('st.multiselect') options = st.multiselect( 'What are your favorite colors', ['Green', 'Yellow', 'Red', 'Blue'], ['Yellow', 'Red']) st.write('You selected:', options)
アプリケーション起動:
% streamlit run day11_st.multiselect.py
起動したブラウザでは以下のような複数選択ウィジェット、プルダウンが表示されました。選択内容に応じて画面下部のJSON表示式も連動して表示が変わっています。
Day 12: st.checkboxの実装
チェックボックスウィジェットを表示するst.checkbox
の実践。
実装コード:
import streamlit as st st.header('st.checkbox') st.write ('What would you like to order?') icecream = st.checkbox('Ice cream') coffee = st.checkbox('Coffee') cola = st.checkbox('Cola') if icecream: st.write("Great! Here's some more 🍦") if coffee: st.write("Okay, here's some coffee ☕") if cola: st.write("Here you go 🥤")
アプリケーション起動:
% streamlit run day12_st.checkbox.py
起動したブラウザでは以下のようなチェックボックスウィジェットが表示されました。任意のチェックボックスを選択するとその選択内容に応じてラベルも表示されています。
Day 13: クラウド開発環境を立ち上げる
Streamlitの開発環境をクラウド上に構築するための手順としてGitPodというサービスの紹介がなされているのがこの13日目。GitHub CodespacesやReplit、Cloud9と同種のサービスとなっているようです。
Day 14: Streamlitコンポーネントの活用
Streamlitで可能なことを拡張するサードパーティのPythonモジュールを「コンポーネント」と呼びます。
StreamlitのWebサイトでは、数十のStreamlit コンポーネントが紹介されており、Streamlit作成者であるFaniloによる「厳選されたStreamlitコンポーネントの一覧」も紹介されています。
利用に際しては必要なライブラリをpipでインストールするだけです。
% pip install streamlit_pandas_profiling
実装コード:
import streamlit as st import pandas as pd import ydata_profiling from streamlit_pandas_profiling import st_profile_report st.header('`streamlit_pandas_profiling`') df = pd.read_csv('https://raw.githubusercontent.com/dataprofessor/data/master/penguins_cleaned.csv') pr = df.profile_report() st_profile_report(pr)
アプリケーション起動:
% streamlit run day14_streamlit-components.py
起動したブラウザでは対処となるCSVデータを読み込んで以下のようなpandasの画面が表示されることを確認出来ました(表示内容的には縦にズラッと並ぶ形になっていたので加工して横に並べています)。コード記述は至ってシンプルですがこれだけでここまでできるんですね。
Day 15: st.latexの実装
LaTeX 形式の数式を表示することができるst.latex
の実装。
実装コード:
import streamlit as st st.header('st.latex') st.latex(r''' a + ar + a r^2 + a r^3 + \cdots + a r^{n-1} = \sum_{k=0}^{n-1} ar^k = a \left(\frac{1-r^{n}}{1-r}\right) ''')
アプリケーション起動:
% streamlit run % streamlit run day15_st.latex.py
起動したブラウザでは以下のような数式が表示されました。
Day 16: Streamlitアプリのテーマのカスタマイズ
.streamlit
フォルダー内のアプリと同じフォルダーに保存されている構成ファイルである「config.toml」のパラメーターを調整することでテーマをカスタマイズできます、という内容の紹介。
実装コード:
import streamlit as st st.title('Customizing the theme of Streamlit apps') st.write('Contents of the `.streamlit/config.toml` file of this app') st.code(""" [theme] primaryColor="#F39C12" backgroundColor="#2E86C1" secondaryBackgroundColor="#AED6F1" textColor="#FFFFFF" font="monospace" """) number = st.sidebar.slider('Select a number:', 0, 10, 5) st.write('Selected number from slider widget is:', number)
設定ファイル:
[theme] primaryColor="#F39C12" backgroundColor="#2E86C1" secondaryBackgroundColor="#7F8C8D" textColor="#FFFFFF" font="monospace"
アプリケーション起動:
% streamlit run day16_style_customized_streamlit_apps.py
起動したブラウザでは以下のような形で(色味に関する)表示内容がカスタマイズされていることを確認出来ました。
Day 17: st.secretsの実装
API キー、データベース パスワード、その他の資格情報などの機密情報を保存できるst.secrets
の実践。.streamlit/secrets.toml
ファイルを用意する仕組みとなっています。
設定ファイルの用意:
OpenAI_key = "your OpenAI key" whitelist = ["sally", "bob", "joe"] [database] user = "!!!USERNAME!!!" password = "!!!PASSWORD!!!"
実装コード:
import streamlit as st st.title('st.secrets') st.write(st.secrets['whitelist']) st.write(st.secrets["database"]["user"]) st.write(st.secrets.database.password)
アプリケーション起動:
% streamlit run day17_st.secrets.py
起動したブラウザでは以下のような形で設定ファイルの内容を参照、取り出すことが出来ました。
Day 18: st.file_uploaderの実装
ファイルアップローダーウィジェットを表示できるst.file_uploader
の実装。
デフォルトでは、アップロードされるファイルは 200MB に制限されているようです。(server.maxUploadSize構成オプションを使用して設定変更可能)
実装コード:
import streamlit as st import pandas as pd st.title('st.file_uploader') st.subheader('Input CSV') uploaded_file = st.file_uploader("Choose a file") if uploaded_file is not None: df = pd.read_csv(uploaded_file) st.subheader('DataFrame') st.write(df) st.subheader('Descriptive Statistics') st.write(df.describe()) else: st.info('☝️ Upload a CSV file')
アプリケーション起動:
% streamlit run day18_st.file_uploader.py
起動したブラウザでは以下のような形でファイルアップロードができる機能が表示されました。
任意のCSVファイル(2024年MLBの成績情報)をアップロードしてみると以下のような形でファイルを表示、解析してくれました。いやーこれは便利ですね。
Day 19: Streamlitアプリをレイアウトする方法
以下コマンドを使ってstreamlitアプリのレイアウト方法について解説されています。
st.set_page_config(layout="wide")
:- アプリのコンテンツをワイドモードで表示(それ以外の場合、デフォルトでは、コンテンツは固定幅のボックスにカプセル化される)
st.sidebar
:- ウィジェットまたはテキスト/画像表示をサイドバーに配置
st.expander
:- 折りたたみ可能なコンテナボックス内にテキスト/画像表示を配置
st.columns
:- コンテンツを配置できる表形式のスペース (または列) を作成
実装コード:
import streamlit as st st.set_page_config(layout="wide") st.title('How to layout your Streamlit app') with st.expander('About this app'): st.write('This app shows the various ways on how you can layout your Streamlit app.') st.image('https://streamlit.io/images/brand/streamlit-logo-secondary-colormark-darktext.png', width=250) st.sidebar.header('Input') user_name = st.sidebar.text_input('What is your name?') user_emoji = st.sidebar.selectbox('Choose an emoji', ['', '😄', '😆', '😊', '😍', '😴', '😕', '😱']) user_food = st.sidebar.selectbox('What is your favorite food?', ['', 'Tom Yum Kung', 'Burrito', 'Lasagna', 'Hamburger', 'Pizza']) st.header('Output') col1, col2, col3 = st.columns(3) with col1: if user_name != '': st.write(f'👋 Hello {user_name}!') else: st.write('👈 Please enter your **name**!') with col2: if user_emoji != '': st.write(f'{user_emoji} is your favorite **emoji**!') else: st.write('👈 Please choose an **emoji**!') with col3: if user_food != '': st.write(f'🍴 **{user_food}** is your favorite **food**!') else: st.write('👈 Please choose your favorite **food**!')
アプリケーション起動:
% streamlit run day19_layout-streamlit-app.py
起動したブラウザでは以下のような形で個別レイアウトされた画面が表示されました。
画面左側のメニューで選択した内容が、画面右側の内容として反映されていることも確認出来ました。
Day 20: Tech Twitter Space on What is Streamlit?
エンジニア/YouTuber/DevRelのFrancesco(@FrancescoCiull4)氏によるTwitterスペースの紹介がなされています。ただ対象となるリンクにアクセスしてみたものの、既にスペースは運用されていないようでした。
まとめ
という訳でStreamlitハンズオン『30 Days of Streamlit』を3日でやってみた、の2日目の実践内容の紹介でした。